>/posts/ones-and-zeros $
Estimated reading time: 14 minutes
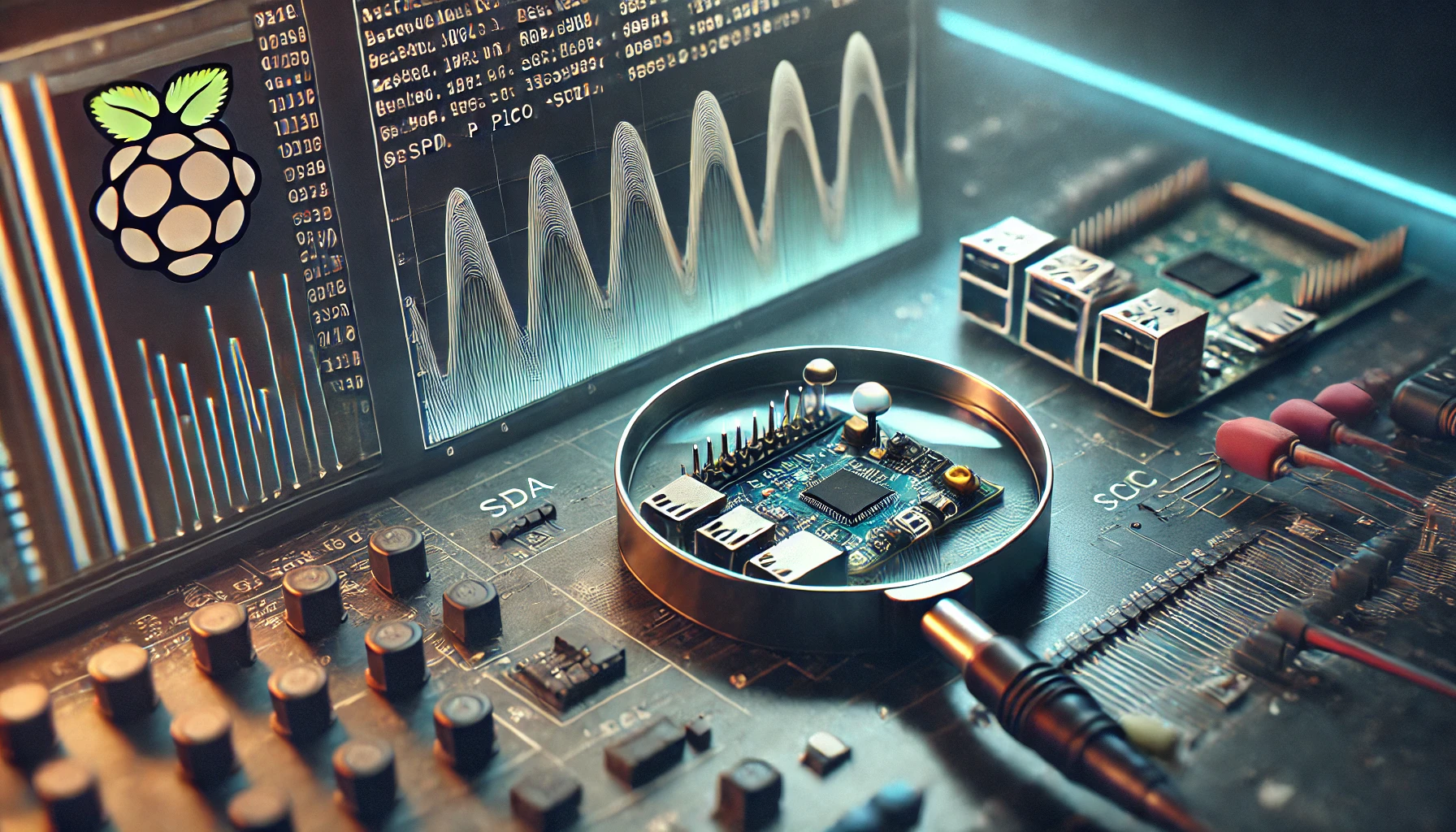
Author: lexu Flag format: ping{.*}
I wanted to see what is displayed on this screen, but i think contrast is too low. Fortunately, you are looking like someone who can read ones and zeroes. I have connected ones and zeroes capture device to the screen and saved the output. Can you help me to read it?
HINT: https://sigrok.org/wiki/File_format:Sigrok/v2
Handout files
What is this task even about?
I started this challenge by taking a look at Discord, as part of my team had already begun working on it. My first glance at the provided charts led me to think -> 2 charts are probably UART or I2C to be decoded.
A quick look at the provided photos -> turns out it’s I2C, the standard protocol for communicating with this display. You could also check which pins on the Raspberry Pi Pico the wires were connected to. Another method was to read the labels "SDA" and "SCL" from the photo of the flipped display, or, as a last resort, you could analyze the provided signal with the data transmission.
A characteristic feature of I2C is that in the idle state (when no one is transmitting), both lines are held HIGH until the master initiates transmission by pulling SDA low. Choose the method that works best for you. (All images are in attachment)
Now, all that’s left is to decode it. A hint was also provided with a link to the "sigrok/v2" documentation, so I downloaded the appropriate software - "PulseView" - and started decoding, but... nothing worked. It turned out that the provided file didn’t give correct results, and it was replaced with a corrected version the next day, so I went back to work.
After loading the corrected file and adding the decoder with the options marked in the screenshot, it was possible to read the I2C commands sent to the display. Now, we can select the option "Export all annotations for this row." We get a file with the following structure:
8319-8460 I2C: Address/data: Address write: 27
8289-8289 I2C: Address/data: Start
8460-8480 I2C: Address/data: Write
8480-8500 I2C: Address/data: ACK
8520-8680 I2C: Address/data: Data write: 0C
8680-8700 I2C: Address/data: ACK
8730-8730 I2C: Address/data: Stop
8765-8765 I2C: Address/data: Start
8795-8936 I2C: Address/data: Address write: 27
8936-8956 I2C: Address/data: Write
8956-8976 I2C: Address/data: ACK
8996-9156 I2C: Address/data: Data write: 08
9156-9176 I2C: Address/data: ACK
9206-9206 I2C: Address/data: Stop
9242-9242 I2C: Address/data: Start
9272-9412 I2C: Address/data: Address write: 27
9412-9432 I2C: Address/data: Write
9432-9452 I2C: Address/data: ACK
9473-9633 I2C: Address/data: Data write: 1C
9633-9653 I2C: Address/data: ACK
9683-9683 I2C: Address/data: Stop
9718-9718 I2C: Address/data: Start
9748-9889 I2C: Address/data: Address write: 27
9889-9909 I2C: Address/data: Write
9909-9929 I2C: Address/data: ACK
This file contains 6000 such lines, but it can be shortened by cutting out only what interests us:
8520-8680 I2C: Address/data: Data write: 0C
8996-9156 I2C: Address/data: Data write: 08
9473-9633 I2C: Address/data: Data write: 1C
9949-10109 I2C: Address/data: Data write: 18
11035-11196 I2C: Address/data: Data write: 0C
11511-11671 I2C: Address/data: Data write: 08
11988-12148 I2C: Address/data: Data write: 2C
12464-12625 I2C: Address/data: Data write: 28
13559-13719 I2C: Address/data: Data write: 7D
14035-14195 I2C: Address/data: Data write: 79
Now it’s only 850 lines, and I eventually reduced it to this form:
"0C,08,1C,18,0C,08,2C,28,7D,79"...
All that’s left is to "just" decode this.
Of course, decoding this manually, including cursor movement and display clearing instructions, is pointless, especially in the era of LLMs (Large Language Models). I sent the display manual "TC1602A-01T.pdf" to Claude.ai — this display is controlled using the I2C protocol — and asked it to write a program that would output the characters displayed on the screen. The code was longer, but I’m only including the most important part with comments explaining what each command does. I also saw on Discord after the competition ended that one of the participants simply sent this data to their own alphanumeric display, which had crossed my mind as well, but unfortunately, I don’t currently have one in my collection of electronic components.
# This method analyzes data where each piece of data is split into 4-bit (nibble) pairs.
# It reads these nibble pairs from an input file and reconstructs them as bytes.
# Then, it extracts the textual (ASCII) portion of the data and returns it as a string.
def analyze_data_4bit(file_path = 'extracted_data.txt')
begin
# Read the entire content of the file.
data = File.read(file_path)
# Split file contents by line, and strip extra whitespace.
clean_lines = data.split("\n").map(&:strip)
# We'll group the lines into pairs of: [control_line, value_line].
pairs = []
clean_lines.each_slice(2) do |control, value|
# Only add a pair if both control and value lines exist (not nil).
pairs << [control, value] if control && value
end
# Convert each line from hexadecimal string to integer (base 16).
# If conversion fails, use 0 by default (rescue).
hex_pairs = pairs.map { |pair| pair.map { |val| val.to_i(16) rescue 0 } }
# This array will hold data about each "transfer" from the LCD’s 4-bit interface.
transfers = []
hex_pairs.each do |control, value|
# rs, rw, and e are specific bits extracted from the control byte.
# (Least significant bit is rs, next is rw, next is e.)
rs = control & 0x01 # Register Select bit
rw = (control >> 1) & 0x01 # Read/Write bit
e = (control >> 2) & 0x01 # Enable bit
# Extract the top 4 bits from the value byte as the data nibble.
data_nibble = (value >> 4) & 0x0F
# Store all of this info as a hash (dictionary) for clarity.
transfers << {
rs: rs,
rw: rw,
e: e,
data: data_nibble,
original: {
control: control,
value: value
}
}
end
# We'll now reconstruct full bytes from pairs of nibbles.
# Typically, LCD data is sent in two nibbles at a time for each byte.
bytes = []
# Loop until the second to last transfer to safely look ahead (i+1).
(0...transfers.size-1).each do |i|
current = transfers[i]
next_transfer = transfers[i+1]
# We assume two consecutive transfers with the same rs/rw combine
# to form a single byte (high nibble + low nibble).
if current[:rs] == next_transfer[:rs] && current[:rw] == next_transfer[:rw]
byte = (current[:data] << 4) | next_transfer[:data]
# We record each reconstructed byte with flags
# to indicate whether it's a command or data.
bytes << {
value: byte,
is_command: current[:rs] == 0,
is_data: current[:rs] == 1
}
end
end
# Finally, we create a string from only those bytes that
# fall into the printable ASCII range (32–126).
data_sequence = bytes
.select { |byte| byte[:is_data] && byte[:value] >= 32 && byte[:value] <= 126 }
.map { |byte| byte[:value].chr } # Convert each ASCII code to its character.
.join
# Return the printable data sequence as a string.
return data_sequence
rescue => e
# If anything goes wrong, we print the error and return nil.
puts "An error occurred: #{e.message}"
puts e.backtrace
return nil
end
end